Exemplos de Programação em Python
Programa Velocímetro
# Programa - Python - Velocímetro
# www.bgmax.com.br/treinamentos
# Necessário instalar a biblioteca plotly
# pip install plotly
# Importa a biblioteca necessária do Plotly para criar gráficos interativos
import plotly.graph_objects as graf
# Cria um objeto figuraura (figura) contendo um velocímetro com cores
figura = graf.Figure(graf.Indicator(
mode="gauge+number+delta", # Modo do indicador (gauge = velocímetro, number = número, delta = diferença)
value=420, # Valor atual do indicador (velocidade)
domain={'x': [0, 1], 'y': [0, 1]}, # Domínio do gráfico (posição e tamanho)
title={'text': "Velocidade", 'font': {'size': 24}}, # Título do indicador
delta={'reference': 400, 'increasing': {'color': "mediumseagreen"}}, # Configuraurações de delta (diferença)
gauge={
'axis': {'range': [0, 500], 'tickwidth': 1, 'tickcolor': "darkblue", 'tickvals': [0, 100, 200, 300, 400, 500]},
# Configuraurações do eixo do velocímetro (intervalo, espessura e cores)
'bar': {'color': "darkblue"}, # Configuraurações da barra do velocímetro (cor)
'bgcolor': "white", # Cor de fundo do velocímetro
'borderwidth': 2, # Largura da borda do velocímetro
'bordercolor': "gray", # Cor da borda do velocímetro
'steps': [
{'range': [0, 100], 'color': 'cyan'},
{'range': [100, 200], 'color': 'deepskyblue'},
{'range': [200, 300], 'color': 'royalblue'},
{'range': [300, 400], 'color': 'cornflowerblue'},
{'range': [400, 500], 'color': 'mediumblue'}
], # Configuraurações dos intervalos de cores no velocímetro
'threshold': {
'line': {'color': "red", 'width': 4},
'thickness': 0.75,
'value': 490
} # Configuraurações da linha de limite (threshold)
}))
# Atualiza o layout da figura (cores de fundo, fonte)
figura.update_layout(
paper_bgcolor="lavender", # Cor de fundo do papel
font={'color': "darkblue", 'family': "Arial"}, # Configuraurações da fonte
annotations=[
{
'x': 0.5,
'y': 0.4,
'xref': 'paper',
'yref': 'paper',
'showarrow': False,
'text': 'Unidades', # Rótulo para as unidades
'font': {'size': 16, 'color': 'darkblue'} # Configuraurações da fonte do rótulo
}
]
)
# Exibe o gráfico
figura.show()
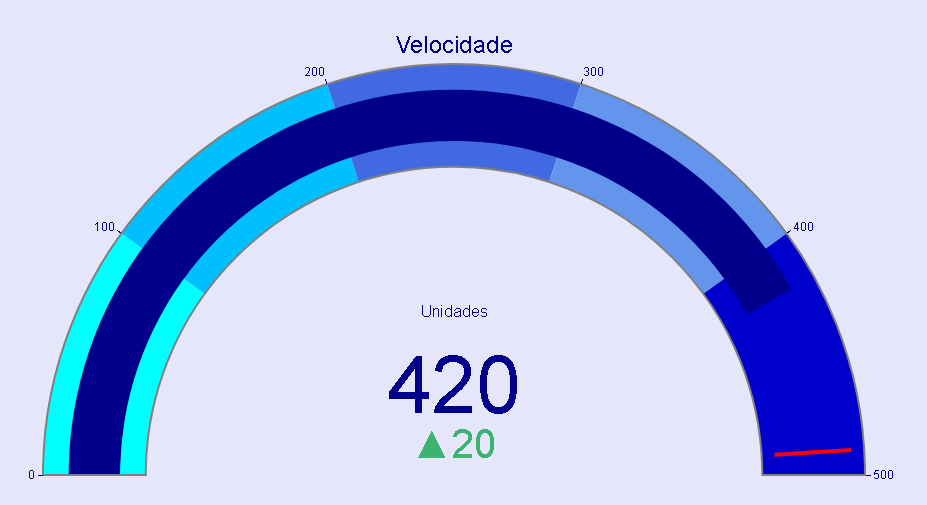
Python + ChatGPT - Inteligência Artificial
# Programa - Python - Python + ChatGPT - Inteligência Artificial
# Desenvolvido por Wilson Borges de Oliveira
# Necessário criar uma chave "API key" no site da OpenAI
# Necessário instalar a bilbioteca openai através do comando abaixo:
# pip install openai
# -*- coding: cp1252 -*-
import tkinter as tk
from tkinter import messagebox
import openai
# Substituir chave aqui
openai.api_key = "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"
def gerar(messages):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=messages,
max_tokens=1024,
temperature=0.5
)
return [response.choices[0].message.content, response.usage]
def preparar():
mensagens = [{"role": "system", "content":
"Você é um assistente gente boa."}]
pergunta = txt_pergunta.get("1.0","end")
mensagens.append({"role": "user", "content": str(pergunta)})
responder = gerar(mensagens)
txt_resposta.config(state= "normal")
txt_resposta.delete("1.0","end")
txt_resposta.insert("1.0",responder[0])
txt_resposta.config(state= "disabled")
mensagens.append({"role": "assistant", "content": responder[0]})
def novo():
txt_pergunta.delete("1.0","end")
txt_resposta.config(state= "normal")
txt_resposta.delete("1.0","end")
def sair():
tela.destroy()
tela = tk.Tk()
tela.geometry('1960x800+0+0')
tela.state('zoomed')
tela.title("Python + ChatGPT - Inteligência Artificial")
tela['bg'] = "yellow"
lbl_pergunta = tk.Label(tela, text ="O que você deseja?", bg="yellow",
fg="black", font=('Calibri', 12, 'bold'), anchor = "w")
lbl_pergunta.place(x = 20, y = 20, height = 25)
txt_pergunta = tk.Text(tela, font=('Calibri', 20), bg="black", fg="white")
txt_pergunta.place(x = 20, y = 50, width=1320, height=70)
btn_gerar = tk.Button(tela, text ="Gerar",
bg ='dimgray',foreground='yellow', font=('Calibri', 14),
command = preparar)
btn_gerar.place(x = 570, y = 150, width = 200, height=60)
lbl_resposta = tk.Label(tela, text ="Resposta:", bg="yellow", fg="black",
font=('Calibri', 12, 'bold'), anchor = "w")
lbl_resposta.place(x = 20, y = 200, height = 25)
txt_resposta = tk.Text(tela, font=('Calibri', 12), bg="black", fg="white")
txt_resposta.place(x = 20, y = 230, width=1300, height=360)
scroll_y = tk.Scrollbar(tela, orient="vertical", command=txt_resposta.yview)
scroll_y.place(x = 1320, y = 230,height=360)
btn_novo = tk.Button(tela, text ="Novo",
bg ='dimgray',foreground='yellow', font=('Calibri', 14),
command = novo)
btn_novo.place(x = 570, y = 620, width = 80, height=40)
btn_sair = tk.Button(tela, text ="Sair",
bg ='dimgray',foreground='yellow', font=('Calibri', 14),
command = sair)
btn_sair.place(x = 690, y = 620, width = 80, height=40)
txt_pergunta.focus_set()
tela.mainloop()
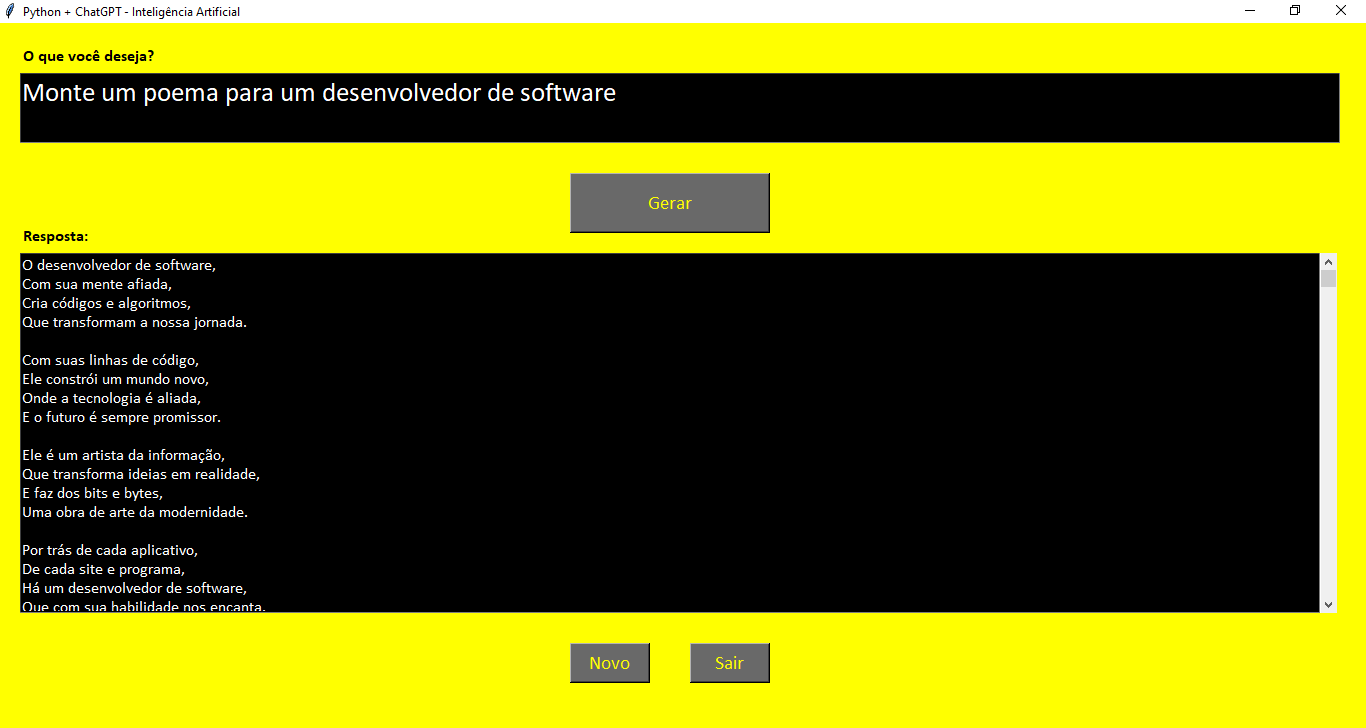
Programa Sorteio da Mega-Sena
# Programa - Sorteio Mega-Sena
# Permite realizar diversos sorteios
# Desenvolvido por Wilson Borges de Oliveira
import random
import tkinter as tk
from tkinter import *
tela = Tk()
tela.title("Sorteio Mega-Sena - Diversos Sorteios")
C = Canvas(tela, bg ="green", height = 600, width = 1000)
C.create_text(500,75, text="Sorteio Mega-Sena",
anchor="c", fill="yellow", font=('Helvetica','22','bold'))
def sorteio():
result = []
random.seed()
while len(result) < 6:
r = random.randint(1, 60)
if r not in result:
result.append(r)
result.sort()
x = 140
y = 200
cont = 0
while cont <6:
oval = C.create_oval(x, y, x + 110, y + 110, fill ="white")
C.create_text(x+52,y+50, text=result[cont],
anchor="c", fill="black", font=('Helvetica','36','bold'))
cont += 1
x += 120
C.pack()
def sair():
tela.destroy()
submitbtn = tk.Button(tela, text ="Novo Sorteio",
bg ='gold',foreground='black', font=('Calibri', 12, 'bold'),
command = sorteio)
submitbtn.place(x = 450, y = 380, width = 150, height=50)
submitbtn = tk.Button(tela, text ="Sair",
bg ='Black',foreground='white', font=('Calibri', 12, 'bold'),
command = sair)
submitbtn.place(x = 820, y = 530, width = 150, height=50)
sorteio()
tela.mainloop()
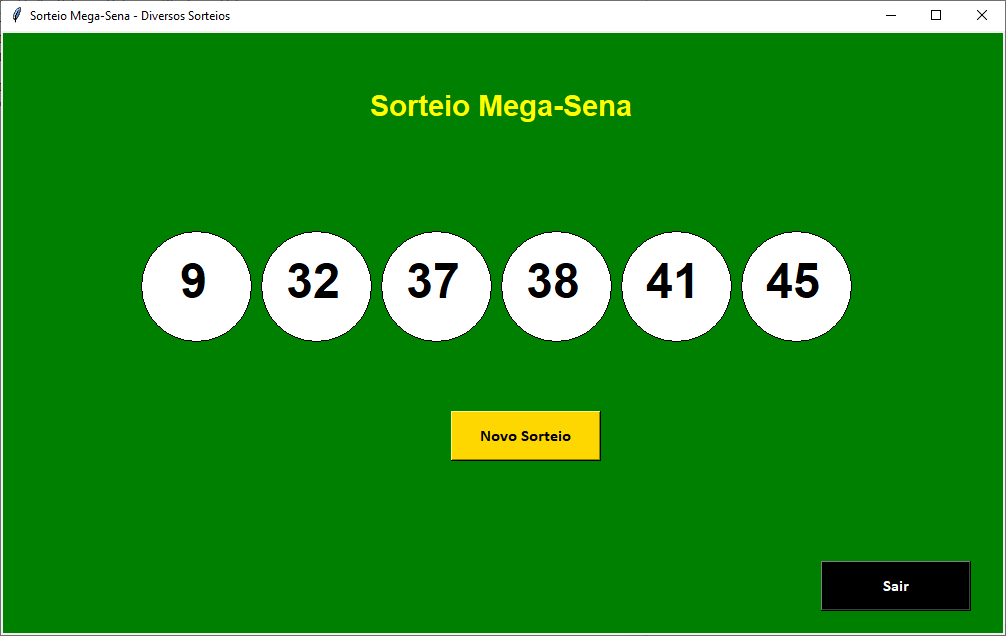
Programa Calcular Área do Retângulo, Círculo e Triângulo
# Programa - Calcular Área do Retângulo, Círculo e Triângulo
# Desenvolvido por Wilson Borges de Oliveira
import math
import tkinter as tk
from tkinter import *
from tkinter import messagebox
tela = Tk()
tela.state('zoomed')
tela.title("Calcular Área do Retângulo, Círculo e Triângulo")
def calc_quadrado():
try:
var_base = float(txt_base_qd.get().replace(',', '.'))
var_altura = float(txt_altura_qd.get().replace(',', '.'))
except:
messagebox.showinfo("Aviso - Retângulo",
"Favor revisar os números digitados")
else:
var_area = var_base * var_altura
txt_area_qd.delete(0,"end")
txt_area_qd.insert(0, f'{var_area:.2f}'.replace('.', ','))
def calc_circulo():
try:
var_raio = float(txt_raio_ci.get().replace(',', '.'))
except:
messagebox.showinfo("Aviso - Círculo",
"Favor revisar os números digitados")
else:
var_area = math.pi * var_raio ** 2
txt_area_ci.delete(0,"end")
txt_area_ci.insert(0, f'{var_area:.2f}'.replace('.', ','))
def calc_triang():
try:
var_base = float(txt_base_tr.get().replace(',', '.'))
var_altura = float(txt_altura_tr.get().replace(',', '.'))
except:
messagebox.showinfo("Aviso - Triângulo",
"Favor revisar os números digitados")
else:
var_area = var_base * var_altura / 2
txt_area_tr.delete(0,"end")
txt_area_tr.insert(0, f'{var_area:.2f}'.replace('.', ','))
C = Canvas(tela, bg ="gold", width = 1960, height = 800)
#Texto
C.create_text(660,35,
text="Calcular Área do Retângulo, Círculo e Triângulo",
anchor="c", fill="black", font=('Helvetica','22','bold'))
#Quadrado
quadradro = C.create_rectangle(100, 100, 300, 300, outline = "black",
fill = "blue", width = 2)
lbl_form_quad = tk.Label(tela, text ="Área = Base × Altura", bg="gold",
fg="black", font=('Calibri', 12, 'bold'))
lbl_form_quad.place(x = 125, y = 310)
lbl_base_qd = tk.Label(tela, text ="Base:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_base_qd.place(x = 125, y = 350, width=90, height = 25)
txt_base_qd = tk.Entry(tela, width = 35, justify=CENTER)
txt_base_qd.place(x = 180, y = 350, width = 75, height = 25)
lbl_altura_qd = tk.Label(tela, text ="Altura:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_altura_qd.place(x = 125, y = 380, width=90, height = 25)
txt_altura_qd = tk.Entry(tela, width = 35, justify=CENTER)
txt_altura_qd.place(x = 180, y = 380, width = 75, height = 25)
btn_quad = tk.Button(tela, text ="Calcular", bg ='yellow',foreground='black',
font=('Calibri', 12, 'bold'), command = calc_quadrado)
btn_quad.place(x = 180, y = 410, width = 75)
lbl_area_qd = tk.Label(tela, text ="Área:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_area_qd.place(x = 125, y = 450, width=90, height = 25)
txt_area_qd = tk.Entry(tela, width = 35, justify=CENTER)
txt_area_qd.place(x = 180, y = 450, width = 75, height = 25)
#Círculo
circulo = C.create_oval(580, 100, 780, 300, outline = "black",
fill ="white", width = 2)
lbl_form_circ = tk.Label(tela, text ="Área = π . r²", bg="gold",
fg="black", font=('Calibri', 12, 'bold'))
lbl_form_circ.place(x = 640, y = 310)
lbl_raio_ci = tk.Label(tela, text ="Raio:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_raio_ci.place(x = 620, y = 350, width=90, height = 25)
txt_raio_ci = tk.Entry(tela, width = 35, justify=CENTER)
txt_raio_ci.place(x = 675, y = 350, width = 75, height = 25)
btn_circ = tk.Button(tela, text ="Calcular", bg ='yellow',foreground='black',
font=('Calibri', 12, 'bold'), command = calc_circulo)
btn_circ.place(x = 675, y = 410, width = 75)
lbl_area_ci = tk.Label(tela, text ="Área:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_area_ci.place(x = 620, y = 450, width=90, height = 25)
txt_area_ci = tk.Entry(tela, width = 35, justify=CENTER)
txt_area_ci.place(x = 675, y = 450, width = 75, height = 25)
#Triângulo
triangulo ={'bounds': [1150, 100, 1050, 300, 1250, 300],
'kind': 'tri', 'fill': True}
C.create_polygon(list(triangulo.values())[0],outline='black',
fill='red', width = 2)
lbl_form_tri2 = tk.Label(tela, text ="___________", bg="gold",
fg="black", font=('Calibri', 12, 'bold'))
lbl_form_tri2.place(x = 1132, y = 307)
lbl_form_tri1 = tk.Label(tela, text ="Área = Base × Altura", bg="gold",
fg="black", font=('Calibri', 12, 'bold'))
lbl_form_tri1.place(x = 1080, y = 302)
lbl_form_circ3 = tk.Label(tela, text ="2", bg="gold", fg="black",
font=('Calibri', 12, 'bold'))
lbl_form_circ3.place(x = 1170, y = 328)
lbl_base_tr = tk.Label(tela, text ="Base:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_base_tr.place(x = 1080, y = 350, width=90, height = 25)
txt_base_tr = tk.Entry(tela, width = 35, justify=CENTER)
txt_base_tr.place(x = 1135, y = 350, width = 75, height = 25)
lbl_altura_tr = tk.Label(tela, text ="Altura:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_altura_tr.place(x = 1080, y = 380, width=90, height = 25)
txt_altura_tr = tk.Entry(tela, width = 35, justify=CENTER)
txt_altura_tr.place(x = 1135, y = 380, width = 75, height = 25)
btn_triang = tk.Button(tela, text ="Calcular", bg ='yellow',foreground='black',
font=('Calibri', 12, 'bold'), command = calc_triang)
btn_triang.place(x = 1135, y = 410, width = 75)
lbl_area_tr = tk.Label(tela, text ="Área:", bg="gold", fg="black",
font=('Calibri', 12), anchor = 'w')
lbl_area_tr.place(x = 1080, y = 450, width=90, height = 25)
txt_area_tr = tk.Entry(tela, width = 35, justify=CENTER)
txt_area_tr.place(x = 1135, y = 450, width = 75, height = 25)
C.pack()
tela.mainloop()
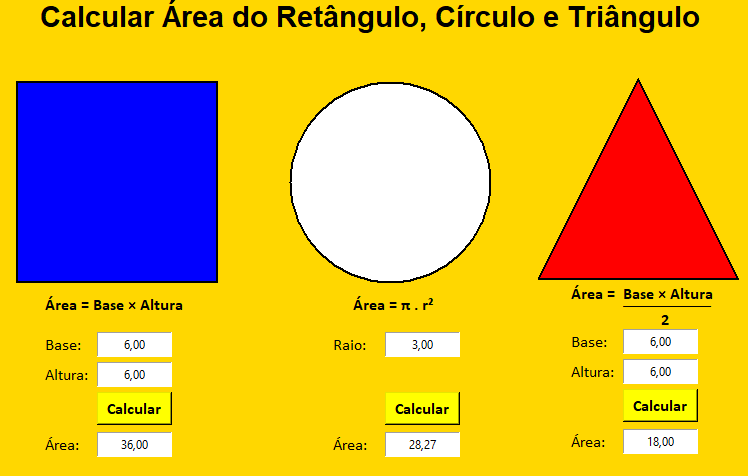
Programa Tabuada com Áudio para Aprendizado Infantil
# Programa Tabuada com Áudio para Aprendizado Infantil
# Desenvolvido por Wilson Borges de Oliveira
# Necessário instalar a biblioteca abaixo:
# pip install pyttsx3
import pyttsx3
engine = pyttsx3.init("sapi5")
engine.setProperty("voice", "brazil")
print ('\n')
n = int(input('Deseja a tabuada de qual número? '))
print ('\n')
for x in range(1,11):
txt_result = str(n) + ' X ' + str(x) + ' = ' + str(x * n)
txt_audio = str(n) + ' vezes ' + str(x) + ' igual a ' + str(x * n)
print(txt_result)
engine.say(txt_audio)
engine.runAndWait()
engine.stop()
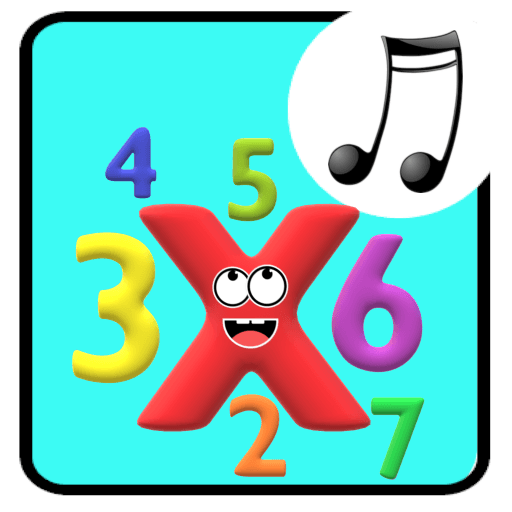
Programa Prisma - Refração da Luz
# Programa Prisma - Refração da Luz
import turtle as caneta
from turtle import Screen
tela = Screen()
tela.title("Prisma - Refração da Luz")
def inicio():
caneta.hideturtle()
caneta.speed('fastest')
caneta.bgcolor('black')
caneta.pencolor('lightblue')
caneta.penup()
caneta.goto(POS_TEXTO)
caneta.write('Prisma - Refração da Luz', True, align='left',font=('Calibri',20,'normal'))
caneta.goto(0,0)
def desenhar_prisma(x,y,lado,cor,largura):
caneta.goto(x,y)
caneta.pendown()
caneta.pencolor(cor)
caneta.pensize(largura)
caneta.backward(lado/2)
caneta.left(60)
caneta.forward(lado)
caneta.right(120)
caneta.forward(lado)
caneta.right(120)
caneta.forward(lado/2)
def desenhar_feixe(x,y,comprimento):
caneta.penup()
caneta.goto(x-comprimento,y+DESLOCAMENTO)
caneta.pendown()
caneta.pencolor('white')
caneta.pensize(15)
caneta.goto(x,y+DESLOCAMENTO)
def desenhar_refracao(cor,x,ate_x,y,ate_y):
caneta.penup()
caneta.goto(x,y + DESLOCAMENTO)
caneta.pendown()
caneta.pencolor(cor)
caneta.pensize(15)
caneta.goto(ate_x,ate_y)
POS_TEXTO = (-130,-190)
DESLOCAMENTO = 60
AZULMAGENTA = '#4B0082'
inicio()
desenhar_prisma(0,-50,200,"white",2)
caneta.speed('slowest')
desenhar_feixe(0,0,400)
desenhar_refracao('red',0,400,0,-80)
desenhar_refracao('orange',0,400,0,-105)
desenhar_refracao('yellow',0,400,0,-130)
desenhar_refracao('green',0,400,0,-155)
desenhar_refracao('mediumblue',0,400,0,-180)
desenhar_refracao('navy',0,400,0,-205)
desenhar_refracao(AZULMAGENTA,0,400,0,-230)
caneta.done()
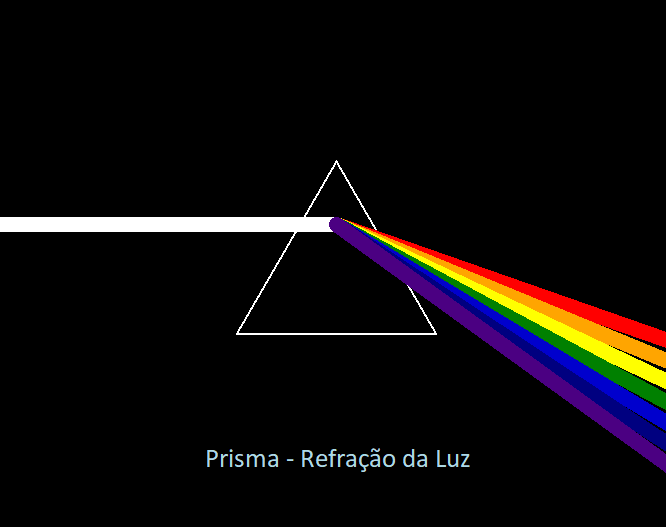
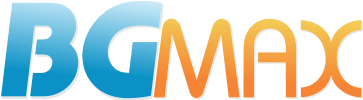
Venha nos ver pessoalmente
Rua Konoi Endo, 33 - Centro
Suzano - SP